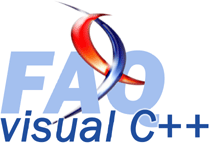
FAQ VC++ et MFCConsultez toutes les FAQ
Nombre d'auteurs : 20, nombre de questions : 545, dernière mise à jour : 5 avril 2013 Ajouter une question
Cette faq a été réalisée pour répondre aux questions les plus fréquement posées sur le forum Développement Visual C++
Je tiens à souligner que cette faq ne garantit en aucun cas que les informations qu'elle contient sont correctes ; Les auteurs font le maximum, mais l'erreur est humaine. Si vous trouvez une erreur, ou si vous souhaitez devenir redacteur, lisez ceci.
Sur ce, je vous souhaite une bonne lecture. Farscape
Il existe deux solutions, l'une pas très propre qui utilise l'interpréteur de commandes (cmd.exe sous WinNt/2000/XP) ainsi que l'opérateur de redirection > et une qui utilise les pipes pour récupérer la sortie standard.
Dans une fenêtre DOS, on peut faire une commande du genre
Code c++ : | Sélectionner tout |
ipconfig /all > result.txt
Pour obtenir le même résultat depuis un programme Windows, on peut soit faire appel à cet interpréteur de command, soit utiliser les pipes pour rediriger la sortie et la récupérer.
1ère Solution :
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | SHELLEXECUTEINFO execinfo; memset(&execinfo, 0, sizeof(execinfo)); execinfo.lpFile = "cmd.exe"; execinfo.cbSize = sizeof(execinfo); execinfo.lpVerb = "open"; execinfo.fMask = SEE_MASK_NOCLOSEPROCESS; execinfo.nShow = SW_SHOWDEFAULT; execinfo.lpParameters = "/c ipconfig.exe /all >> result.txt"; if (!ShellExecuteEx(&execinfo)) { LPVOID lpMsgBuf; FormatMessage(FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM | FORMAT_MESSAGE_IGNORE_INSERTS, NULL, GetLastError(), 0, (LPTSTR) &lpMsgBuf, 0, NULL); MessageBox(NULL,(LPCTSTR)lpMsgBuf,"",MB_ICONSTOP); LocalFree( lpMsgBuf ); } WaitForSingleObject(execinfo.hProcess, INFINITE); |
2ième Solution:
Voici une fonction qui permet de faire une redirection
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | #define BUFFER 4096 bool executeCmdWithRedirection(char * command, char * fileDest) { SECURITY_DESCRIPTOR sd; SECURITY_ATTRIBUTES sa; InitializeSecurityDescriptor(&sd, SECURITY_DESCRIPTOR_REVISION); SetSecurityDescriptorDacl(&sd, true, NULL, false); sa.nLength = sizeof(SECURITY_ATTRIBUTES); sa.bInheritHandle = true; sa.lpSecurityDescriptor = &sd; HANDLE hReadPipe; HANDLE hWritePipe; if (! CreatePipe(&hReadPipe, &hWritePipe, &sa, NULL)) return false; STARTUPINFO si; memset(&si, 0, sizeof(STARTUPINFO)); si.cb = sizeof(STARTUPINFO); si.dwFlags = STARTF_USESHOWWINDOW |STARTF_USESTDHANDLES; si.wShowWindow = SW_SHOW; si.hStdOutput = hWritePipe; si.hStdError = hWritePipe; PROCESS_INFORMATION pi; if ( ! CreateProcess(NULL, command, NULL, NULL, TRUE, 0, 0, 0, &si, &api) ) return false; WaitForSingleObject(pi.hProcess, INFINITE); HANDLE hFichier; hFichier = CreateFile(fileDest, GENERIC_WRITE, NULL, NULL, CREATE_NEW, FILE_ATTRIBUTE_NORMAL, NULL); if( hFichier == INVALID_HANDLE_VALUE) return false; DWORD BytesRead ; DWORD lu; char dest[BUFFER]; memset(dest, 0, BUFFER); DWORD byteAvail = 0; PeekNamedPipe(hReadPipe, NULL, 0, NULL, &byteAvail, NULL); if (!byteAvail) // la génération n'a rien produit return true; while (ReadFile(hReadPipe, dest, BUFFER, &BytesRead, NULL)) { if (!BytesRead) break; WriteFile(hFichier, dest, BytesRead, &lu, NULL); PeekNamedPipe(hReadPipe, NULL, 0, NULL, &byteAvail, NULL); if (BytesRead<BUFFER || !byteAvail) break; } CloseHandle(hFichier); CloseHandle(hReadPipe); CloseHandle(hWritePipe); return true; } |
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 | if (executeCmdWithRedirection("ipconfig.exe /all", "sortie.txt")) { /* OK*/ } else { /* erreur*/ } |
Les Apis 32 fournissent toutes les fonctionnalités pour gérer une application en mode console.
L'exemple suivant est une classe permettant la gestion de l'écran et du clavier avec le support de la couleur.
Code C++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 | #include <conio.h> class CCsleWin { public: CCsleWin() { if(!m_nCountCsle) AllocConsole(); m_nCountCsle++; m_hStdOut=m_hStdIn=NULL; m_hStdOut = GetStdHandle(STD_OUTPUT_HANDLE); m_hStdIn = GetStdHandle(STD_INPUT_HANDLE); SetConsoleMode(m_hStdIn,ENABLE_WINDOW_INPUT); m_co.X=m_co.Y=0; m_Color=7; } // ------------------- virtual ~CCsleWin() { CloseHandle(m_hStdIn); CloseHandle(m_hStdOut); m_hStdOut=NULL; m_hStdIn=NULL; m_nCountCsle--; if(!m_nCountCsle) FreeConsole(); } // ------------------- // fixe la taille en hauteur et largeur de la console. void SetSize(int nWidth=80,int nHeight=25) { m_co.X=nWidth; m_co.Y=nHeight; if(!m_hStdOut) return ; SetConsoleScreenBufferSize(m_hStdOut, m_co); ClearScreen(); } // ------------------- // renvoie la largeur et la hauteur en nb de caracteres de la console. void GetSize(int &rnWidth,int &rnHeight) { rnWidth=m_co.X;rnHeight=m_co.Y; } // ------------------- // renvoie la largeur en nb de caracteres de la console int GetWidth() { int nWidth,nHeight; GetSize(nWidth,nHeight); return nWidth; } // ------------------- // renvoie la hauteur en nb de caracteres de la console int GetHeight() { int nWidth,nHeight; GetSize(nWidth,nHeight); return nHeight; } // ------------------ // position le curseur a la colonne nx et la ligne ny void GotoXY(int nx=0,int ny=0) { COORD co={ny,nx}; if(!m_hStdOut) return; SetConsoleCursorPosition(m_hStdOut,co); } // ------------------- // renvoie les coordonnées colonne et ligne du curseur void GetCursorPos(int &rnx,int &rny) { CONSOLE_SCREEN_BUFFER_INFO csbiInfo; rnx=rny=0; if(!m_hStdOut) return ; if (! GetConsoleScreenBufferInfo(m_hStdOut, &csbiInfo)) return; rnx=csbiInfo.dwCursorPosition.X; rny=csbiInfo.dwCursorPosition.Y; } // ------------------- // renvoie la colonne du curseur int GetXPos() { int x,y;GetCursorPos(x,y);return x; } // ------------------- // renvoie la ligne du curseur int GetYPos() { int x,y;GetCursorPos(x,y);return y; } // lecture des caracteres a l'ecran ,renvoie un buffer // liberation a la charge de l'utilisateur. // ----------------------------------- char *ReadScreen(int nLig,int nCol,int nWidth,int nHeight) { char *pCarBuf= new char [nWidth*nWidth]; if(!ReadScreen(nLig,nCol,nWidth,nHeight,pCarBuf)) { delete []pCarBuf; return NULL; } return pCarBuf; } // ----------------------------------- bool ReadScreen(int nLig,int nCol,int nWidth,int nHeight,char *pbuff) { DWORD cCharsWritten; COORD coord; if(!m_hStdOut) return false; coord.Y=nLig;coord.X=nCol; return(ReadConsoleOutputCharacter(m_hStdOut, pbuff, nWidth*nHeight, coord, &cCharsWritten)==TRUE); } // ----------------------------------- // lecture des attributs couleurs ,renvoie un tableau de word . // liberation a la charge de l'utilisateur. WORD *ReadScreenAtt(int nLig,int nCol,int nWidth,int nHeight) { WORD *pCarBufAtt= new WORD [nWidth*nHeight]; if(!ReadScreenAtt(nLig,nCol,nWidth,nHeight,pCarBufAtt)) { delete []pCarBufAtt; return NULL; } return pCarBufAtt; } // ----------------------------------- bool ReadScreenAtt(int nLig,int nCol,int nWidth,int nHeight,WORD *pbuff) { DWORD cCharsWritten; COORD coord; if(!m_hStdOut) return false; coord.Y=nLig;coord.X=nCol; return(ReadConsoleOutputAttribute(m_hStdOut, pbuff, nWidth*nHeight, coord, &cCharsWritten)==TRUE); } // ------------------- // fait un srcoll de l'ecran. void ScrollLine() { if(!m_hStdOut) return ; CONSOLE_SCREEN_BUFFER_INFO csbiInfo; if (! GetConsoleScreenBufferInfo(m_hStdOut, &csbiInfo)) return; csbiInfo.dwCursorPosition.X = 0; // If it is the last line in the screen buffer, scroll // the buffer up. if ((csbiInfo.dwSize.Y-1) == csbiInfo.dwCursorPosition.Y) { SMALL_RECT srctWindow; if (csbiInfo.srWindow.Top > 0) { srctWindow.Top = -1; // move top up by one row srctWindow.Bottom = -1; // move bottom up by one row srctWindow.Left = 0; // no change srctWindow.Right = 0; // no change if (! SetConsoleWindowInfo( m_hStdOut, // screen buffer handle FALSE, // deltas, not absolute &srctWindow)) // specifies new location { return; } } else // { // scrolling par lecture des informations ecran. char *pCarBuf= new char [GetWidth()*GetHeight()]; WORD *pCarBufAtt= new WORD [GetWidth()*GetHeight()]; if(ReadScreen(0,0,GetWidth(),GetHeight(),pCarBuf) && ReadScreenAtt(0,0,GetWidth(),GetHeight(),pCarBufAtt)) { COORD coord={0,0}; DWORD cCharsWritten; memcpy(pCarBuf,pCarBuf+GetWidth(), (GetWidth()*GetHeight())-GetWidth()); memcpy(pCarBufAtt, ((char *)pCarBufAtt)+(GetWidth()*sizeof(WORD)), (GetWidth()*GetHeight())-GetWidth()*sizeof(WORD)); memset(pCarBuf+(GetWidth()*GetHeight())-GetWidth(),' ',GetWidth()); memset(((char *)pCarBufAtt)+(((GetWidth()*GetHeight())-GetWidth())*sizeof(WORD)), (WORD)GetColor(),GetWidth()*sizeof(WORD)); WriteConsoleOutputCharacter(m_hStdOut, pCarBuf, GetWidth()*GetHeight(), coord, &cCharsWritten); WriteConsoleOutputAttribute(m_hStdOut, pCarBufAtt, GetWidth()*GetHeight(), coord, &cCharsWritten); } delete []pCarBuf; delete []pCarBufAtt; } } // Otherwise, advance the cursor to the next line. else csbiInfo.dwCursorPosition.Y += 1; if (! SetConsoleCursorPosition(m_hStdOut,csbiInfo.dwCursorPosition)) { return; } } // ------------------- // primitive d'affichage d'une chaîne avec couleur courante. bool MessString(const char *szMess,bool bSetColor=false,int nLenght=0) { DWORD cCharsWritten; int nx,ny,nlen; if(!m_hStdOut) return false; nlen=(nLenght?nLenght:strlen(szMess)); if(bSetColor) GetCursorPos(nx,ny); WriteConsole(m_hStdOut, szMess, nlen, &cCharsWritten, NULL); if(bSetColor) FillRgnColor(ny,nx,nlen,1,GetColor()); return (cCharsWritten==(unsigned )nlen); } // ------------------------------- // affichage d'un caractere bool PutCh(int c) { char sz[2]; sprintf(sz,"%c",c); return MessString(sz); } // ------------------------------- // affichage d'une chaîne avec gestion de la couleur bool CPuts(const char *pString) { return MessString(pString,true); } // ------------------------------- // affichage formatté avec gestion de la couleur bool CPrintf(const char *fmt, ...) { if(!m_hStdOut) return false; char *psz= new char [GetWidth()+1]; va_list argptr; int cnt; va_start(argptr, fmt); cnt = vsprintf(psz, fmt, argptr); va_end(argptr); bool bOk=MessString(psz,true); delete []psz; return bOk; } // -------------------------------- // affichage formatté bool Printf(const char *fmt, ...) { if(!m_hStdOut) return false; char *psz= new char [GetWidth()+1]; va_list argptr; int cnt; va_start(argptr, fmt); cnt = vsprintf(psz, fmt, argptr); va_end(argptr); bool bOk=MessString(psz); delete []psz; return bOk; } // ------------------- // effacer l'écran. void ClearScreen() { FillRgnColor(0,0,m_co.Y,m_co.X,0,' '); GotoXY(); } // ------------------- // fixe la couleur en cours void SetColor(int nColor){m_Color=nColor;} // ------------------- // renvoie la couleur en cours. int GetColor(){return m_Color;} // ------------------- // change la couleur pour une surface void FillRgnColor(int nLig,int nCol,int nWidth,int nHeight, int nColor,char nChar=0) { COORD coord; if(!m_hStdOut) return; for(int nlig=nLig;nlig<nLig+nHeight;nlig++) { DWORD cWritten; coord.Y=nlig; coord.X=nCol; FillConsoleOutputAttribute( m_hStdOut, nColor, nWidth, coord, &cWritten); if(nChar) FillConsoleOutputCharacter(m_hStdOut, (TCHAR)nChar, (DWORD)nWidth, coord, &cWritten); } } // ------------------- // primitive de lecture clavier voir documentation MSDN: "Virtual key codes" pour la definition des touches int GetCh() { DWORD i; int nCarCode; struct EVENT_KEYBOARD EventKeyBoard; bool bOk; if(!m_hStdIn) return 0; bOk=false; while(!bOk) { ReadConsoleInput(EventKeyBoard); for(i=0;i<EventKeyBoard.cRead;i++) { switch(EventKeyBoard.EventType) { case KEY_EVENT: if(EventKeyBoard.wVirtualKeyCode==16) continue;// shift if(EventKeyBoard.wVirtualKeyCode==17) continue;// ctrl if(EventKeyBoard.wVirtualKeyCode==18) continue;// alt if(EventKeyBoard.bKeyDown) { if(EventKeyBoard.AsciiChar) nCarCode=EventKeyBoard.AsciiChar; else nCarCode=EventKeyBoard.wVirtualKeyCode; bOk=true; } break; case MOUSE_EVENT: continue; case WINDOW_BUFFER_SIZE_EVENT: continue; case MENU_EVENT: continue; case FOCUS_EVENT: continue; } } } return(nCarCode); } private: struct EVENT_KEYBOARD { WORD EventType; BOOL bKeyDown; WORD wVirtualKeyCode; DWORD dwControlKeyState; unsigned char AsciiChar; WORD wVirtualScanCode; DWORD cRead; }; void ReadConsoleInput(struct EVENT_KEYBOARD &rEventKeyBoard) { INPUT_RECORD irInBuf[1]; ::ReadConsoleInput(m_hStdIn,irInBuf,1,&rEventKeyBoard.cRead); rEventKeyBoard.EventType=irInBuf[0].EventType; rEventKeyBoard.bKeyDown=irInBuf[0].Event.KeyEvent.bKeyDown; rEventKeyBoard.wVirtualKeyCode=irInBuf[0].Event.KeyEvent.wVirtualKeyCode; rEventKeyBoard.dwControlKeyState=irInBuf[0].Event.KeyEvent.dwControlKeyState; rEventKeyBoard.AsciiChar=irInBuf[0].Event.KeyEvent.uChar.AsciiChar; rEventKeyBoard.wVirtualScanCode=irInBuf[0].Event.KeyEvent.wVirtualScanCode; } private: HANDLE m_hStdOut,m_hStdIn; static int m_nCountCsle; COORDm_co; intm_Color; }; int CCsleWin::m_nCountCsle = 0; int main(int argc, char* argv[]) { CCsleWin Csle; Csle.SetSize(); Csle.SetColor(78); Csle.MessString("Hello World!\n",true); int n; Csle.GotoXY(10,0); do { n=Csle.GetCh(); if((Csle.GetXPos()==Csle.GetWidth()-1) || (Csle.GetYPos()==Csle.GetHeight()-1 && Csle.GetXPos()==Csle.GetWidth()-1)) Csle.ScrollLine(); Csle.Printf("%c",n); } while(n!=27); return 0; } |
Le Programme complet : console.zip
Note : Les fonctions de cette classe sont inline pour des raisons pratiques d'écriture.
On se sert des API CharToOem et OemToChar pour les conversions.
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 | char maChaine[20] = "abcdéùàabc"; printf("%s\n",maChaine); // afiche abcdÚ¨Óabc CharToOem(maChaine,maChaine); printf("%s\n",maChaine); // affiche abcdéùàabc char saisie[20]; scanf("%s",saisie); // saisir abcdéùàabc printf("%s\n",saisie); // affiche abcdéùàabc OemToChar(saisie,saisie); printf("%s\n",saisie); // afiche abcdÚ¨Óabc |
En unicode, on ne peut bien sur pas faire de même, car la taille de la chaîne est double (caractères codés sur deux octets) => il faut donc utiliser deux variables.
Pour les CString, le principe est le même, sachant que les CString disposent de méthodes membres : AnsiToOemet OemToAnsi
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 | CString chaine = "abcùàéabc"; printf("%s\n",chaine); chaine.AnsiToOem(); printf("%s\n",chaine); scanf("%s",chaine); printf("%s\n",chaine); chaine.OemToAnsi(); printf("%s\n",chaine); |
On peut se servir de la simulation des touches ALT+ENTREE
Code c++ : | Sélectionner tout |
1 2 3 4 5 6 7 | void altEntree() { keybd_event(VK_MENU,0x38,0,0); keybd_event(VK_RETURN,0x1c,0,0); keybd_event(VK_RETURN,0x1c,KEYEVENTF_KEYUP,0); keybd_event(VK_MENU,0x38,KEYEVENTF_KEYUP,0); } |
En utilisant les fonctions win32 dédiées au mode console.
L'exemple ci-dessous montre l'implémentation d'une fenêtre de trace debug en mode console, avec le support éventuel d'un fichier trace .
Code C++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | // classe d'application class CMyApp : public CWinApp { public: CMyApp (); ~ CMyApp (); // Start trace debug void StartDebugConsole(int nWidth,int nHeight,const char* pszfname=NULL); // Line feed void DebugNewLine(void); // Use DebugPrintf like TRACE0, TRACE1, ... (The arguments are the same as printf) voidDebugPrintf(const char *szfmt,...); public: HANDLE m_hStdOut; CStdioFile m_stdFileDebug; // Overrides // ClassWizard generated virtual function overrides //{{AFX_VIRTUAL(CMyApp) public: virtual BOOL InitInstance(); //}}AFX_VIRTUAL // Implementation //{{AFX_MSG(CMyApp) afx_msg void OnAppAbout(); // NOTE - the ClassWizard will add and remove member functions here. // DO NOT EDIT what you see in these blocks of generated code ! //}}AFX_MSG DECLARE_MESSAGE_MAP() }; |
Code C++ : | Sélectionner tout |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | CMyApp::CMyApp () { m_hStdOut=NULL; } CMyApp::~CMyApp() { // Destruct #ifdef _DEBUG if(m_stdFileDebug.m_pStream != NULL) m_stdFileDebug.Close(); if(m_hStdOut) { FreeConsole(); CloseHandle(m_hStdOut); } #endif } //------------------------------------------------------------------- void CMyApp::StartDebugConsole(int nWidth, int nHeight,const char* pszfname) { #ifdef _DEBUG if(m_hStdOut) return; AllocConsole(); SetConsoleTitle("Debug Window"); m_hStdOut = GetStdHandle(STD_OUTPUT_HANDLE); COORD co = {nWidth, nHeight }; SetConsoleScreenBufferSize(m_hStdOut, co); if(pszfname) m_stdFileDebug.Open(pszfname, CFile::modeCreate |CFile::modeReadWrite |CFile::typeText |CFile::shareDenyWrite); co.X=co.Y = 0; SetConsoleCursorPosition(m_hStdOut,co); #endif } //------------------------------------------------------------------- void CMyApp::DebugNewLine(void) { #ifdef _DEBUG CONSOLE_SCREEN_BUFFER_INFO csbiInfo; if (! GetConsoleScreenBufferInfo(m_hStdOut, &csbiInfo)) return; csbiInfo.dwCursorPosition.X = 0; // If it is the last line in the screen buffer, scroll // the buffer up. if ((csbiInfo.dwSize.Y-1) == csbiInfo.dwCursorPosition.Y) { SMALL_RECT srctWindow; if(csbiInfo.srWindow.Top > 0) { srctWindow.Top = -1; // move top up by one row srctWindow.Bottom = -1; // move bottom up by one row srctWindow.Left = 0; // no change srctWindow.Right = 0; // no change if (! SetConsoleWindowInfo( m_hStdOut, // screen buffer handle FALSE, // deltas, not absolute &srctWindow)) // specifies new location { return; } } } // Otherwise, advance the cursor to the next line. else csbiInfo.dwCursorPosition.Y += 1; if (! SetConsoleCursorPosition(m_hStdOut, csbiInfo.dwCursorPosition)) { return; } #endif } //------------------------------------------------------------------- // Use DebugPrintf like TRACE0, TRACE1, ... (The arguments are the same as printf) void CMyApp::DebugPrintf(const char *szfmt, ...) { #ifdef _DEBUG char s[300]; va_list argptr; int cnt; va_start(argptr, szfmt); cnt = vsprintf(s, szfmt, argptr); va_end(argptr); DWORD cCharsWritten; if(m_hStdOut) { DebugNewLine(); WriteConsole(m_hStdOut, s, strlen(s), &cCharsWritten, NULL); } if(m_stdFileDebug.m_pStream!=NULL) { CString str=s; str+="\r\n"; m_stdFileDebug.WriteString(str); } #endif } |
Utilisation à partir d'un endroit quelconque du programme :
Code C++ : | Sélectionner tout |
1 2 3 | ((CMyApp *)AfxGetApp())->StartDebugConsole(80,25,"essai.txt"); for(int i=0;i<5;i++) ((CMyApp *)AfxGetApp())->DebugPrintf("Txt Ligne:%d",i); |
Exemple d'une classe console complète : console.zip
Proposer une nouvelle réponse sur la FAQ
Ce n'est pas l'endroit pour poser des questions, allez plutôt sur le forum de la rubrique pour çaLes sources présentées sur cette page sont libres de droits et vous pouvez les utiliser à votre convenance. Par contre, la page de présentation constitue une œuvre intellectuelle protégée par les droits d'auteur. Copyright © 2025 Developpez Developpez LLC. Tous droits réservés Developpez LLC. Aucune reproduction, même partielle, ne peut être faite de ce site et de l'ensemble de son contenu : textes, documents et images sans l'autorisation expresse de Developpez LLC. Sinon vous encourez selon la loi jusqu'à trois ans de prison et jusqu'à 300 000 € de dommages et intérêts.